Jetpack Compose VS SwiftUI !VS Flutter

Android development has become easier as the updates keep on coming. After the 2020.3.1 update, a lot has changed. But the main change which I think most of the developers must be waiting for is the Jetpack Compose for the production application.

And Kotlin is the only option for jetpack Compose, which is also the preferred language.
For more details or changes on Jetpack Compose can go to https://developer.android.com/jetpack/compose.
Similarly, iOS Development also provides an option for declarative development, SwiftUI. In IDE, there was no change due to this.
But the concept is almost the same as Jetpack Compose. Instead of storyboard, we create UI using Swift.

For more details or changes on SwiftUI go to https://developer.apple.com/xcode/swiftui/
Let’s see how both of them work using a demo project. I have taken a similar number of taps example of Flutter.
Android Jetpack Compose
We can create UI using composable, and they can be divided into smaller parts for re-useability.
Let’s create a Text view that shows the number of taps, which takes an Integer as an input.
@Composable
fun Taps(taps: Int) {
Text(
text = "$taps",
style = MaterialTheme.typography.h1
)
}
Now an Add Button will allow the user to tap on to increase the count which takes a function onTap which will be called on every tap.
@Composable
fun AddButton(onTap: () -> Unit) {
FloatingActionButton(
onClick = onTap,
) {
Icon(Icons.Filled.Add, "")
}
}
Finally create the main composable which will contain all the above views and other views to make up the whole screen UI.
@Composable
fun MyApp(tapViewModel: TapViewModel = viewModel()) {
val taps: Int by tapViewModel.taps.observeAsState(0)
Scaffold(
topBar = {
TopAppBar(
title = { Text("Jetpack Compose Demo Home Page") },
backgroundColor = MaterialTheme.colors.primaryVariant,
contentColor = Color.White
)
},
floatingActionButton = {
AddButton(
onTap = {
tapViewModel.onTap()
}
)
},
content = {
Column(
modifier = Modifier.fillMaxSize(),
verticalArrangement = Arrangement.Center,
horizontalAlignment = Alignment.CenterHorizontally,
) {
Text(
"You have tapped the button this many times:",
textAlign = TextAlign.Center
)
Taps(taps = taps)
}
},
)
}
Till now whatever we have done will create the UI but nothing will happen when the button is clicked. So for that, we will use the TapViewModel class which will hold our application state.
/// A view model which will be used to update the UIclass TapViewModel : ViewModel() {
private val _taps = MutableLiveData(0)
val taps: LiveData<Int> = _taps // A method which will be called to update the tap value
fun onTap() {
_taps.value = _taps.value?.plus(1)
}
}
Now, we have our UI and state management. So let’s put it on the screen.
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
NumberOfClicksTheme {
Surface(color = MaterialTheme.colors.background) {
MyApp()
}
}
}
}
}
Everything is done from the code side. Let’s run and check if all the functionality is working as expected or not.
As you can see the functionality is what we expected. Now let me put my thoughts as Advantages and Disadvantages.
Advantages:
- Fast development.
- Better UI reusability.
- Better state management.
- Easy to understand code.
- No separate XML file is required for UI.
Disadvantages:
- Still new in the market.
- For now, fewer resources to learn from.
- For new developers, it may take little time to understand the structure. (Might be, it’s just a personal opinion).
- Breaking changes might occur.
But altogether it’s good to start the Android Application Development using Compose. Because as the time goes many more features will be added into this. And the best part is, it can also be added to your existing applications.
Check out the below link for WHY Compose? https://developer.android.com/jetpack/compose/why-adopt

SwiftUI
Let’s make a similar application for iOS using SwiftUI. The concept is the same as any other declarative UI framework. In this also the UI can be divided into smaller views.
I have added the code below which is required to make the same number of tap application,
struct ContentView: View {
@State var taps = 0
var body: some View {
NavigationView {
ZStack {
VStack {
Text("You have tapped the button this many times:")
.navigationBarTitle("SwiftUI Demo Home Page") Text("\(taps)")
.font(.system(size: 30))
.fontWeight(.bold)
}
ZStack(alignment: .bottomTrailing) {
Rectangle()
.foregroundColor(.clear)
.frame(maxWidth: .infinity, maxHeight: .infinity)
Button(action: {
self.taps += 1
}) {
Image(systemName: "plus.circle.fill")
.resizable()
.frame(width: 75, height: 75)
.foregroundColor(Color(red: 153/255, green: 102/255, blue: 255/255))
.shadow(color: .gray, radius: 0.2, x: 1, y: 1)
}
}.padding()
}
}
.ignoresSafeArea()
}
}
This is all you need to make the number of tap applications in SwiftUI. Now let’s see how the output looks like,
As you can see, the expected output is the same as the Jetpack Compose. Now let me put my thoughts as Advantages and Disadvantages.
Advantages:
- Less code.
- No StoryBoard connection is required.
- Faster development.
- Easy to understand.
- Better code division.
- Better screen size optimization.
Disadvantages:
- Still pretty new.
- Breaking changes might occur.
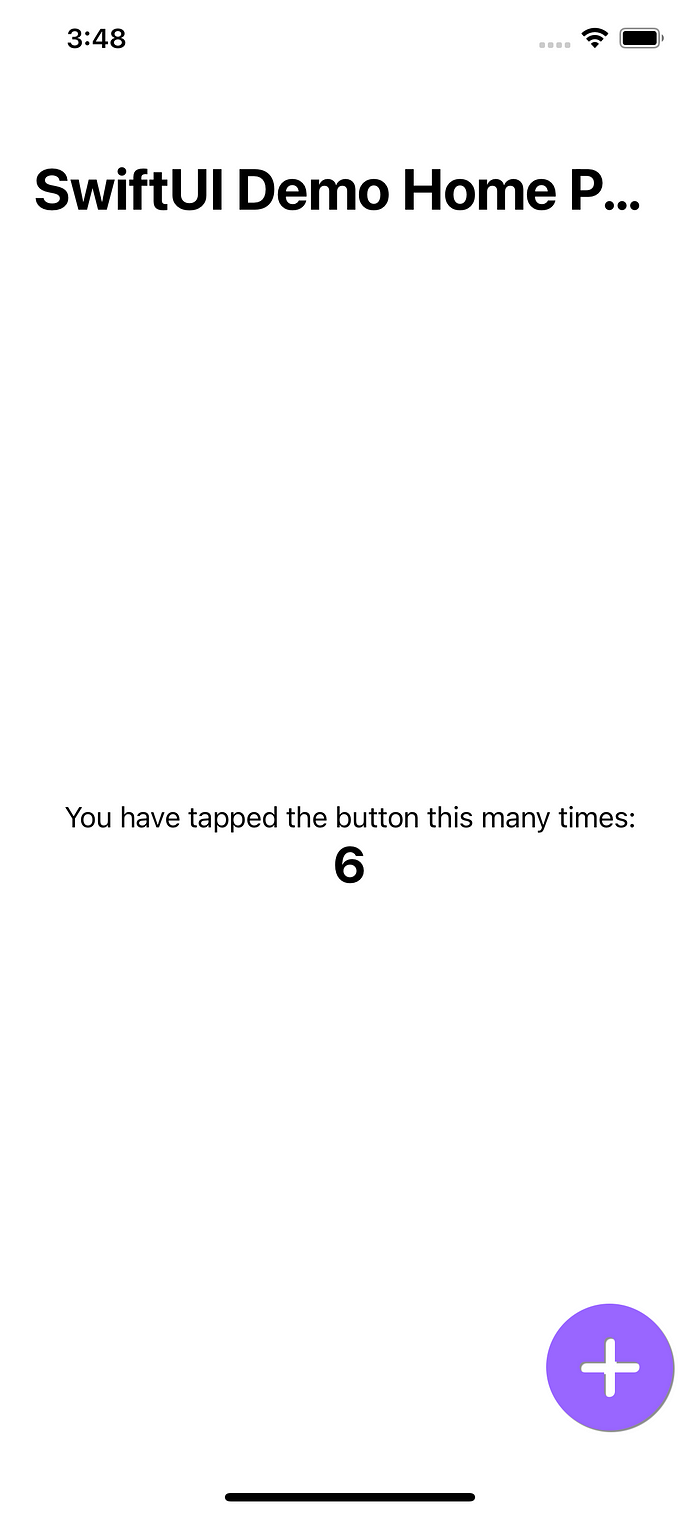
As you can see, the advantages and disadvantages are almost similar for both. And it will be because the concept is almost the same with name changes. And according to be declarative UI is the future of development, because every development framework is moving towards that.
And as the title suggests I am not comparing these two with Flutter. Jetpack Compose & SwiftUI is much more different than Flutter. Both are for native development and Flutter is for cross-platform. Even though the structure & concept is almost the same still they can’t be compared with each other.
For all the code access go to https://github.com/pradyotprksh/JetpackComposeSwiftUi
The code which you see in the repository shouldn’t be taken as a way of using these frameworks. There are more better ways provided by other developers. This article is just for comparing my personal opinion on Jetpack Compose and SwiftUI. 😇